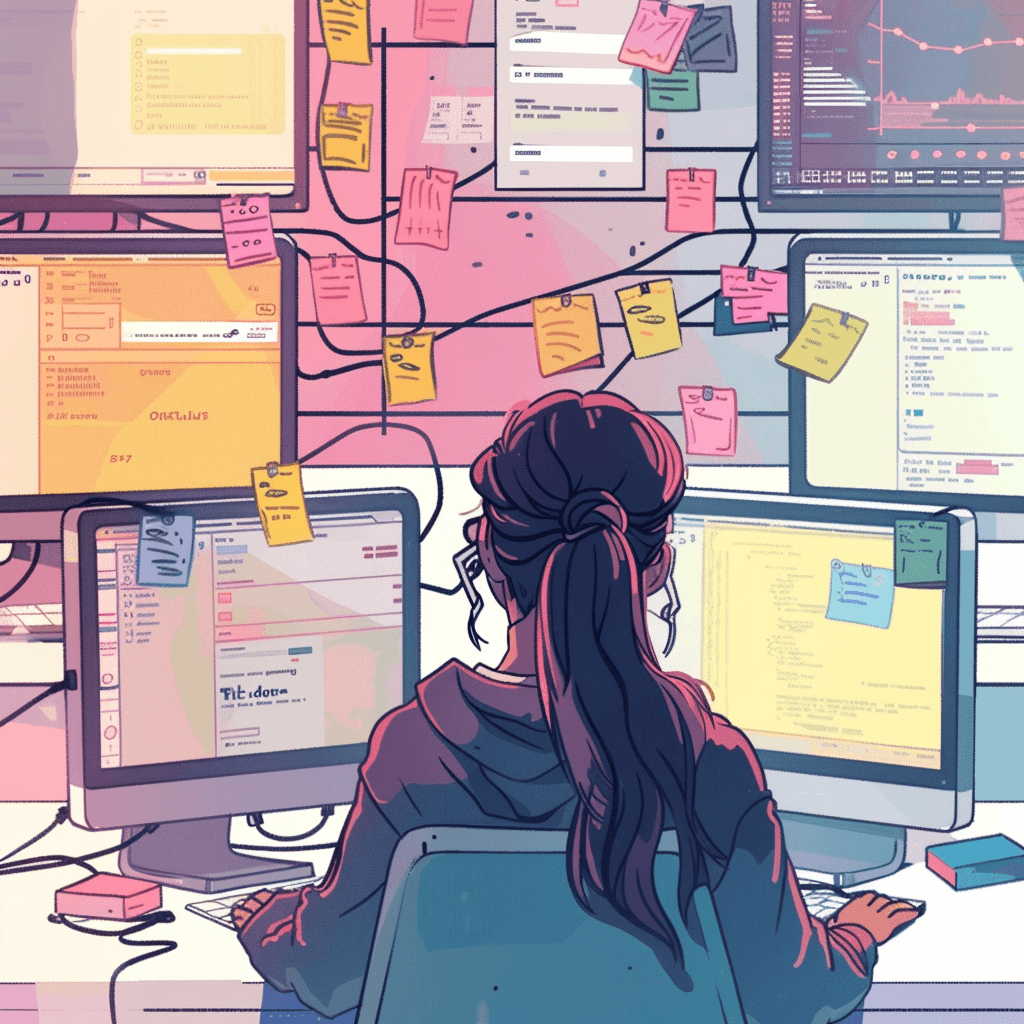
Introduction
Coding can be fun, challenging, and at times, hair-pullingly frustrating. No matter your state of mind while coding, you’ll often want to jump back and forth between alternative solutions—whether to compare, find bugs, or simply figure out how that piece of code you copied from Stack Overflow is supposed to work.
When first starting out it is quite common to go the route of a gazillion documents with similar names “test.cs”, “test_2.cs”, “final_test.cs”, “ok_now_it_should_work.cs”, “LKFJDGLKAJGLKAJ.cs” etc
While this approach can work, it has many flaws. It’s easy to get lost between the files and forget which file contained which solution. Moreover, sharing code among a group of developers is nearly impossible using this system.
Enter Git!
Git is a version control system that’s essential for managing code changes, collaborating with teammates, and maintaining the integrity of your projects. Think of Git as a time machine for your code—allowing you to track, revert, and experiment without fear. On top of that, it lets you share code with others in smart and efficient ways.
Let’s dive into the what, why, and how of Git to get you started on the right foot.
What is Git?
Git is a distributed version control system that lets you track changes to your files and coordinate work on those files among multiple people. It was created by Linus Torvalds in 2005 and has since become the de facto standard for version control in the software industry. Unlike centralized version control systems (VCS) that rely on a single server to store all versions of a project’s files, Git allows every developer to have a complete copy of the repository. This makes it faster and more reliable, especially when working offline or in environments with unreliable network connectivity.
There are other version control systems you might encounter depending on your workplace or tech stack, but Git’s principles will help you adapt to any of them.
Why Use Git?
1. History and Backup: Git keeps a detailed history of changes, allowing you to revert to previous states if something goes wrong. Essentially, you create save points while coding, making it possible to load previous “saves” if necessary. Each save (commit) can contain changes to any number of files, so you don’t have to remember which versions of different files go together.
One of the beauties of Git (and all version control systems) is that Git only saves one version of each file and complements that with a history that records the changes. So, if you want two versions of a file with only one line different, you don’t create an entirely new file but instead keep the file and save the change to the history.
2. Collaboration: Multiple developers can work on the same project simultaneously without overwriting each other’s changes. Each developer has a local repository containing all the project’s files and their history. The team or project has one common central repository, commonly referred to as the remote repository, which is updated by individual developers when they want to share their work.
3. Branching and Merging: Git’s branching model enables you to work on different features or bug fixes in isolation and merge them back into the main project when ready. Just as each developer has their own repository with a local history, a developer can set up multiple “parallel histories,” known as branches.
Instead of making copies of all your files and putting them in a separate folder, Git allows you to create a new “history-file.” Whenever you want, you can switch between branches, and Git will update your code based on that branch’s history.
Branches can be shared between developers using the central repository, facilitating collaboration on features or bug fixes. Developer 1 pushes (uploads) their local branch to the Remote (central) repository, developer 2 pulls (downloads) it to their local computer and now Dev 2 can do whatever they need to do on their machine without it affecting the code on Dev 1’s computer.
4. Experimentation: You can create branches to try out new ideas without affecting the stable codebase. Branches are commonly used for various purposes, including feature development, bug fixes, and experiments.
A great benefit of branches for experimentation is that you can work with all the existing code without fear of breaking something for another developer or messing up what’s currently in production. If the experiment doesn’t pan out, you can discard the branch and create a new one from the main branch (trunk).
How to Use Git
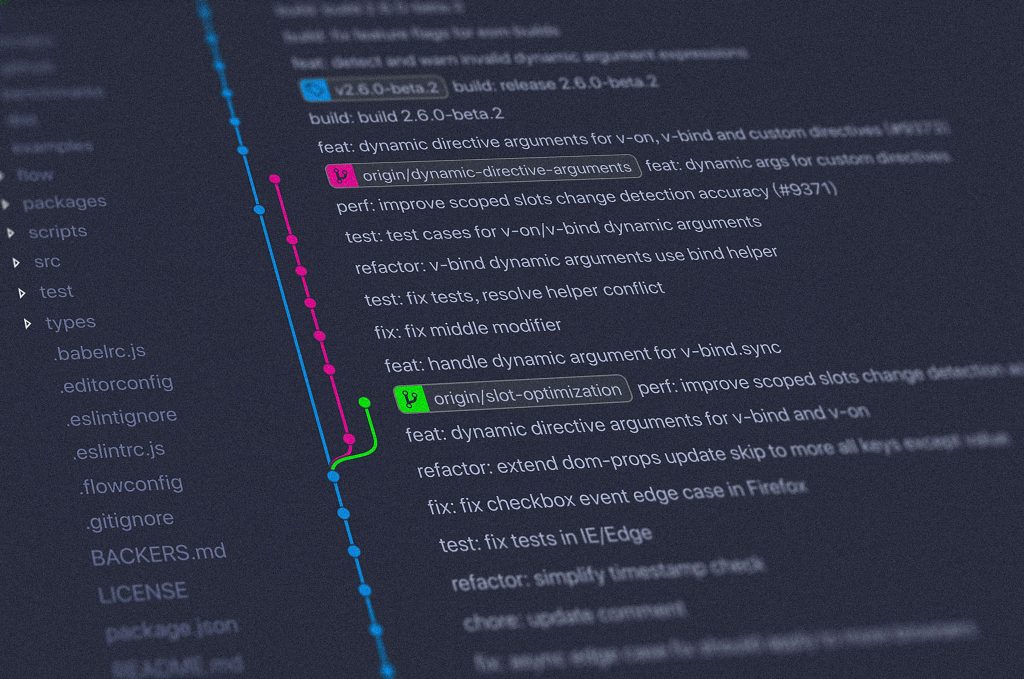
(Photo by Yancy Min)
Basic Concepts
– Repository (Repo): A Git repository is a directory that contains your project files and the entire history of their changes.
– Commit: A snapshot of your project files at a particular point in time.
– Branch: A parallel version of your project where you can make changes without affecting the main codebase (often called `main` or `master`, which is often considered the trunk of the “code tree”).
– Merge: Combining changes from one branch into another.
– Remote: A version of your repository hosted on the internet or another network.
Getting Started with Git
1. Installing Git: If you haven’t already, download and install Git from [git-scm.com](https://git-scm.com/).
2. Setting Up Git: Configure your Git environment with your name and email.
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
3. Initializing a Repository: Navigate to your project directory and initialize a Git repository.
git init
Basic Git Commands
1. Clone a Repository: Copy an existing Git repository to your local machine.
git clone <repository-url>
2. Check Status: See the status of your working directory.
git status
3. **Add Changes**: Stage changes for the next commit.
git add <file> # Add a specific file
git add . # Add all changes
4. Commit Changes: Save your staged changes with a descriptive message.
git commit -m "Add feature X"
Alternatively, if you want to commit with title and description, simply use the -m flag two times:
git commit -m <title> -m <description>
If you wan your description to span multiple lines (e.g when making a list of changes) you can press enter before the second “.
git commit -m "A big change" "1. My first change
> 2. My second change
> 3. My third change"
The commit message won’t end until you add the closing quote. [Stack Overflow]
5. Push Changes: Upload your local commits to a remote repository.
git push origin <branch>
6. Pull Changes: Fetch and merge changes from a remote repository.
git pull
Visual Git Tools
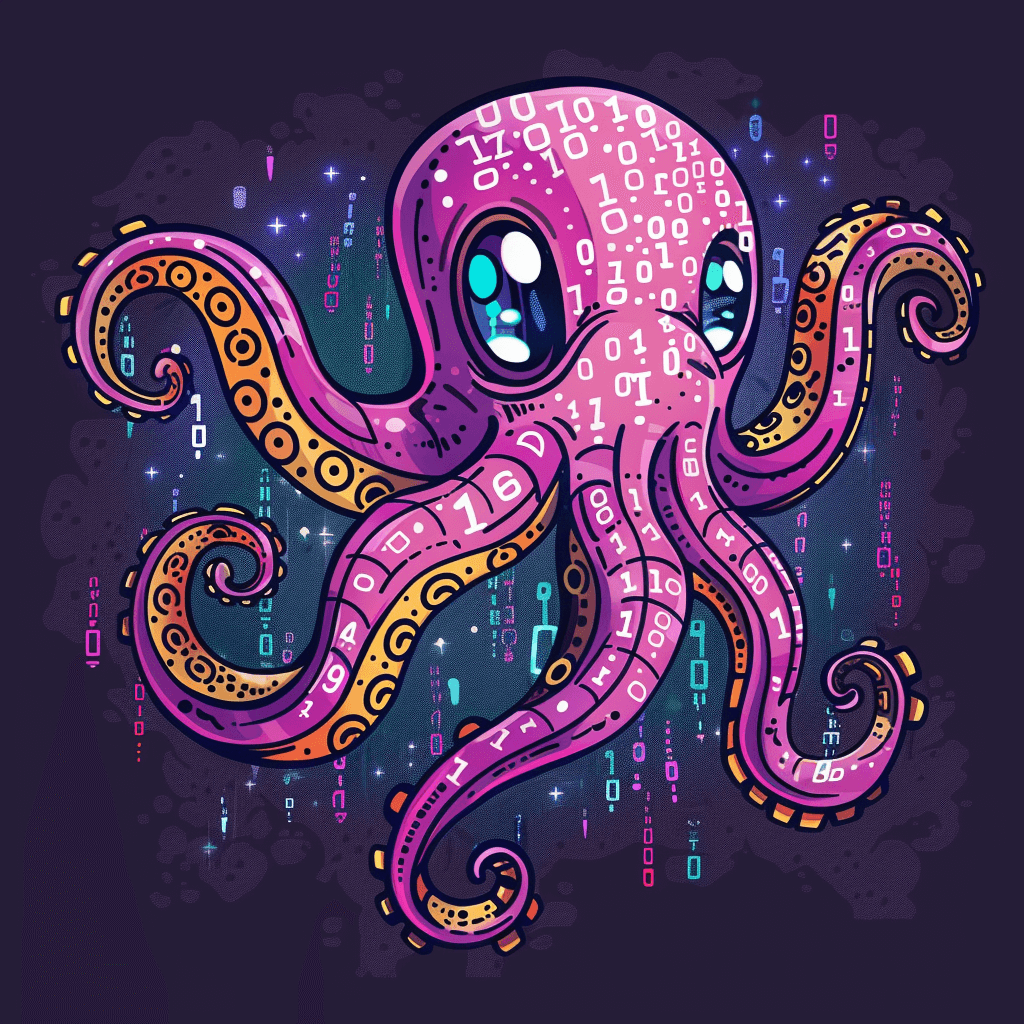
While mastering Git commands in the terminal is invaluable, visual Git tools like GitKraken and GitHub Desktop can significantly streamline your workflow, especially when you’re getting started. GitKraken offers an intuitive interface with powerful features such as a visually appealing commit graph, drag-and-drop functionality for merging branches, and an integrated code editor, making it easier to manage complex repositories. On the other hand, GitHub Desktop provides a seamless experience for those who frequently use GitHub, with features like simple synchronization of local and remote repositories, straightforward conflict resolution, and easy access to pull requests and issues. These tools not only make Git more accessible but also enhance productivity by providing visual context to your version control processes. Whether you’re a novice or a seasoned developer, incorporating these tools can help you navigate Git’s complexities with greater ease and confidence.
Working with Branches
Branches are crucial for managing different lines of development. Here’s how to think about them:
– Main Branch: This is your stable production-ready branch.
– Feature Branches: Create a new branch for each feature or bug fix.
git checkout -b <feature-branch>
– Merging Branches: Once your feature is complete, merge it back into the main branch.
git checkout main
git merge <feature-branch>
– Deleting Branches: After merging, you can delete the feature branch.
git branch -d <feature-branch>
Best Practices
1. Create a new branch for every feature, bug fix, experiment etc: By encapsulating each feature within its own branch it will be much easier to figure out what works and what doesn’t. Avoid mixing multiple features, bug fixes etc within the same branch since it is harder to review by others and to debug if something goes wrong.
2. Commit Often: Make small, frequent commits with clear messages. This makes it easier to track changes and identify issues.
3. Write Good Commit Messages: A good commit message is concise yet descriptive. Use the format:
Short summary (50 characters or less)
Detailed explanation (if necessary, 72 characters per line)
4. Stay in Sync: Regularly pull changes from the remote repository to stay updated with your team’s progress.
5. Resolve Conflicts Promptly: If you encounter merge conflicts, address them as soon as possible to avoid complications.
6. Review Code: Use pull requests or code reviews to ensure quality and consistency before merging changes into the main branch.
7. Avoid Merge Conflicts: When working with a team of developers, try to work on different parts of the code base at any given time in order to avoid merge conflicts (they appear when you have made different changes to the same line of code).
Collaborating with Others
When working with others, communication is key. Here are some tips for effective collaboration:
– Use Pull Requests: These allow others to review your changes before they are merged.
– Keep Your Branch Up-to-Date: Regularly rebase or merge the main branch into your feature branch to minimize conflicts.
– Communicate: Let your team know what you’re working on and if you need help.
Conclusion
Git might seem overwhelming at first, but with practice, it will become an invaluable part of your workflow. Remember, it’s not just about using Git commands but understanding the principles behind them. By following best practices and maintaining clear communication, you’ll find that Git greatly enhances your ability to manage and collaborate on projects effectively.
The article above might give you the impression that there are a bunch of rules and A Correct Way Of Doing ThingsTM when it comes to Git and version control. The swell thing is that in most cases it is not such a big deal if you diverge from best practices! Commit messages can be amended (updated), if you put multiple features into the same branch you can try to break them apart, or simply learn from your mistakes and make future branches more focused.
It is always better to try and fail than to not try at all for fear of angering highly opinionated people.
So go forth and enjoy the fruits of the Git tree, because they are yummy!
Further Reading
Articles and Tutorials
- Atlassian Git Tutorial: A comprehensive guide covering Git basics, branching, merging, and advanced topics.
- Git – The Simple Guide: A concise, straightforward guide to getting started with Git.
- How to Write Better Git Commit Messages – A Step-By-Step Guide: A good primer with explanations and examples
- Git Immersion: A guided tour that walks you through the fundamentals of Git, with hands-on labs and examples.
Free Ebooks
- Pro Git: As mentioned, this is one of the best comprehensive resources available for free. Covers both basic and advanced Git concepts.
- Learn Version Control with Git: A free ebook by Tower, covering Git basics in an easy-to-understand format.
Videos and Online Courses
- Udacity Git and GitHub Course: A free course that covers Git and GitHub essentials.
- GitHub Docs & Guides: Official GitHub documentation, guides and tutorials to help you get started with GitHub.
Cheat Sheets
- Git Cheat Sheet by GitHub: A handy PDF covering essential Git commands and workflows.
- Atlassian Git Cheat Sheet: Another useful cheat sheet from Atlassian, providing a quick reference to common Git commands.